Data Tagging
Make sure Bluetooth is enabled on your phone and authorized to Ecotopia App. Follow below steps to tag the data.
Step 1:
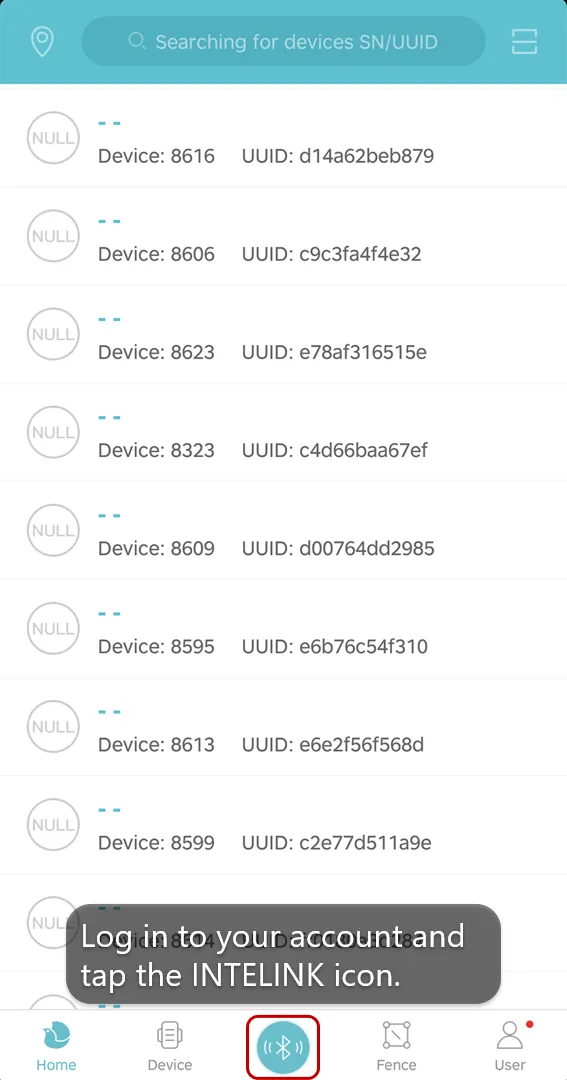
Step 2:
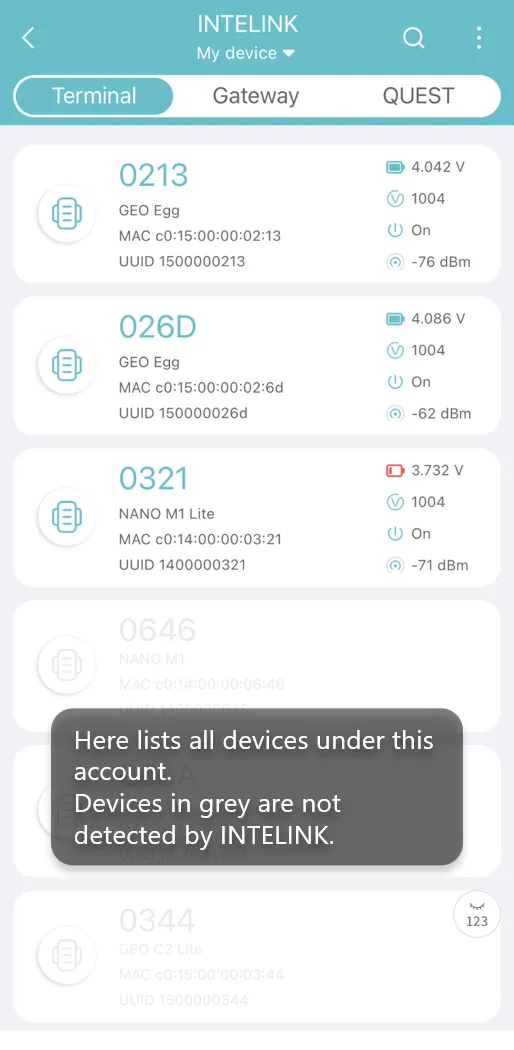
Step 3:
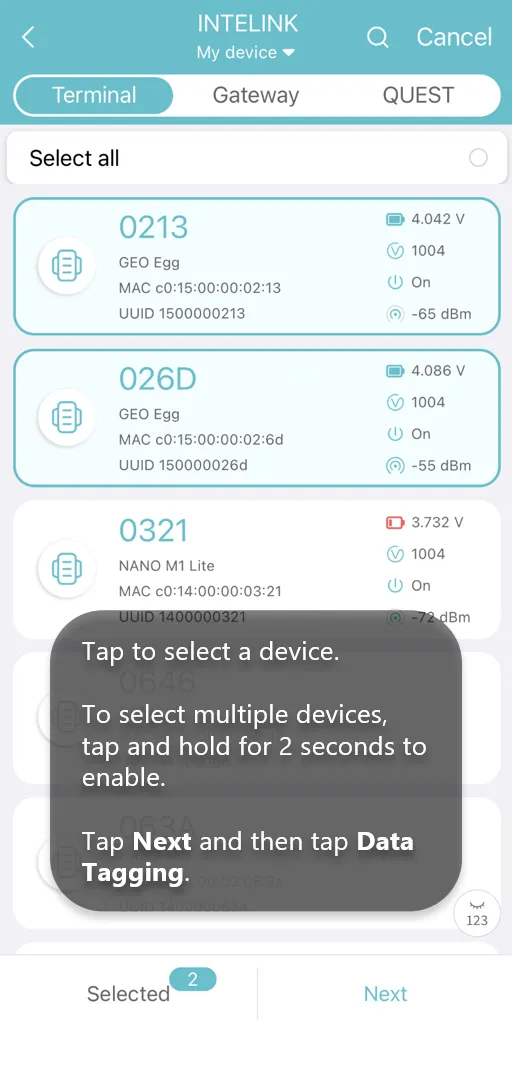
Step 4:
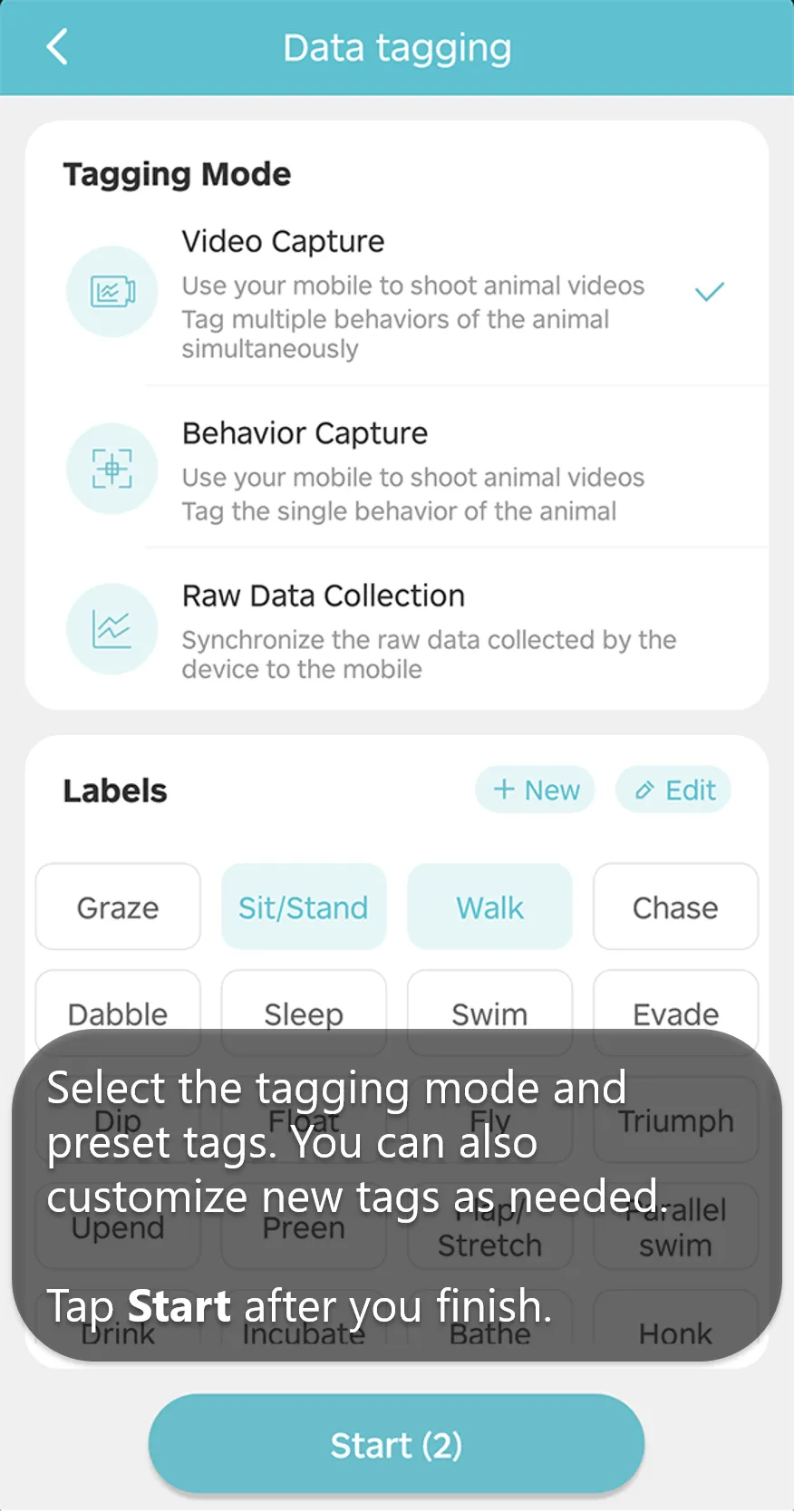
Step 5:
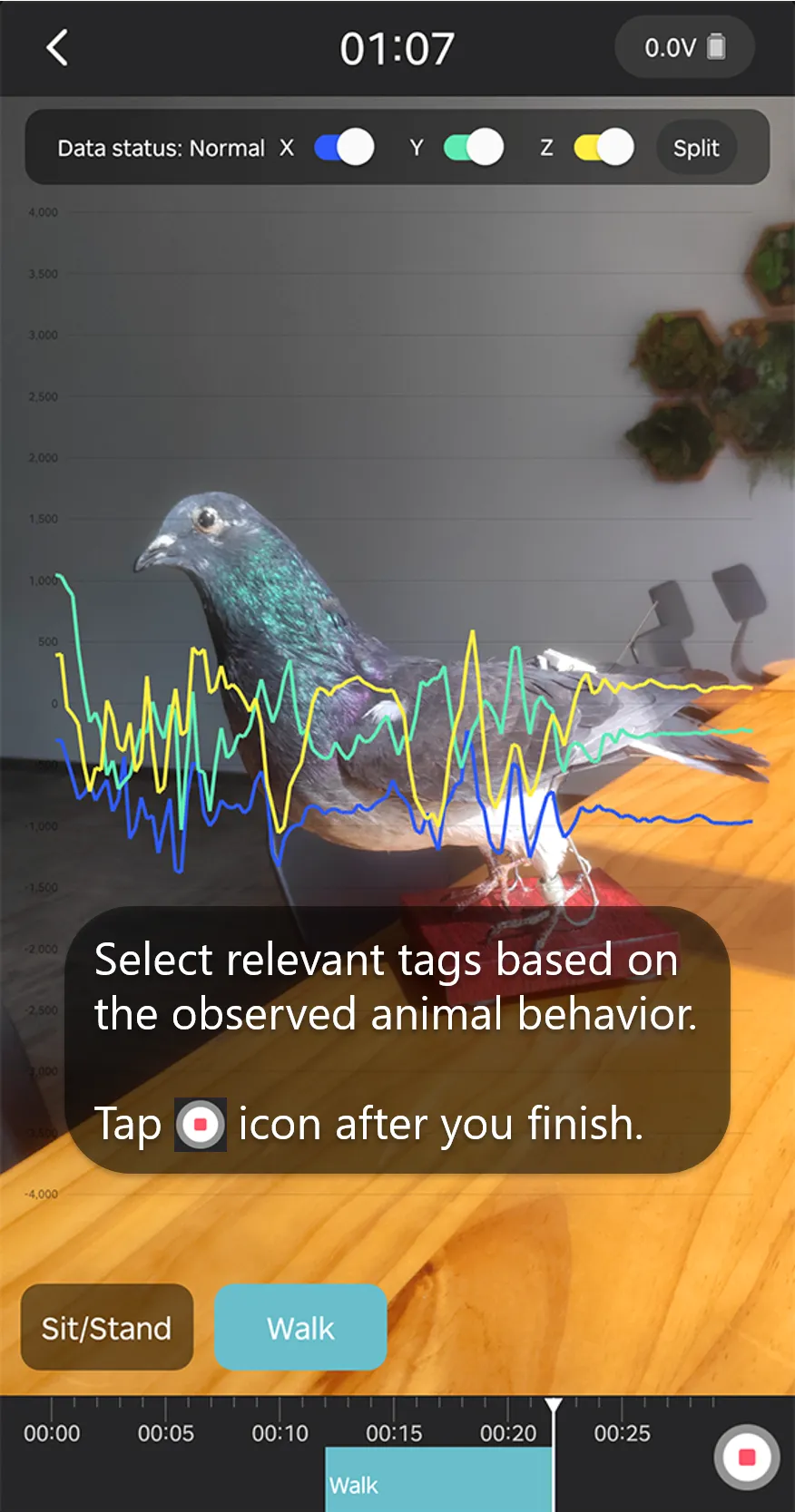
Step 6:
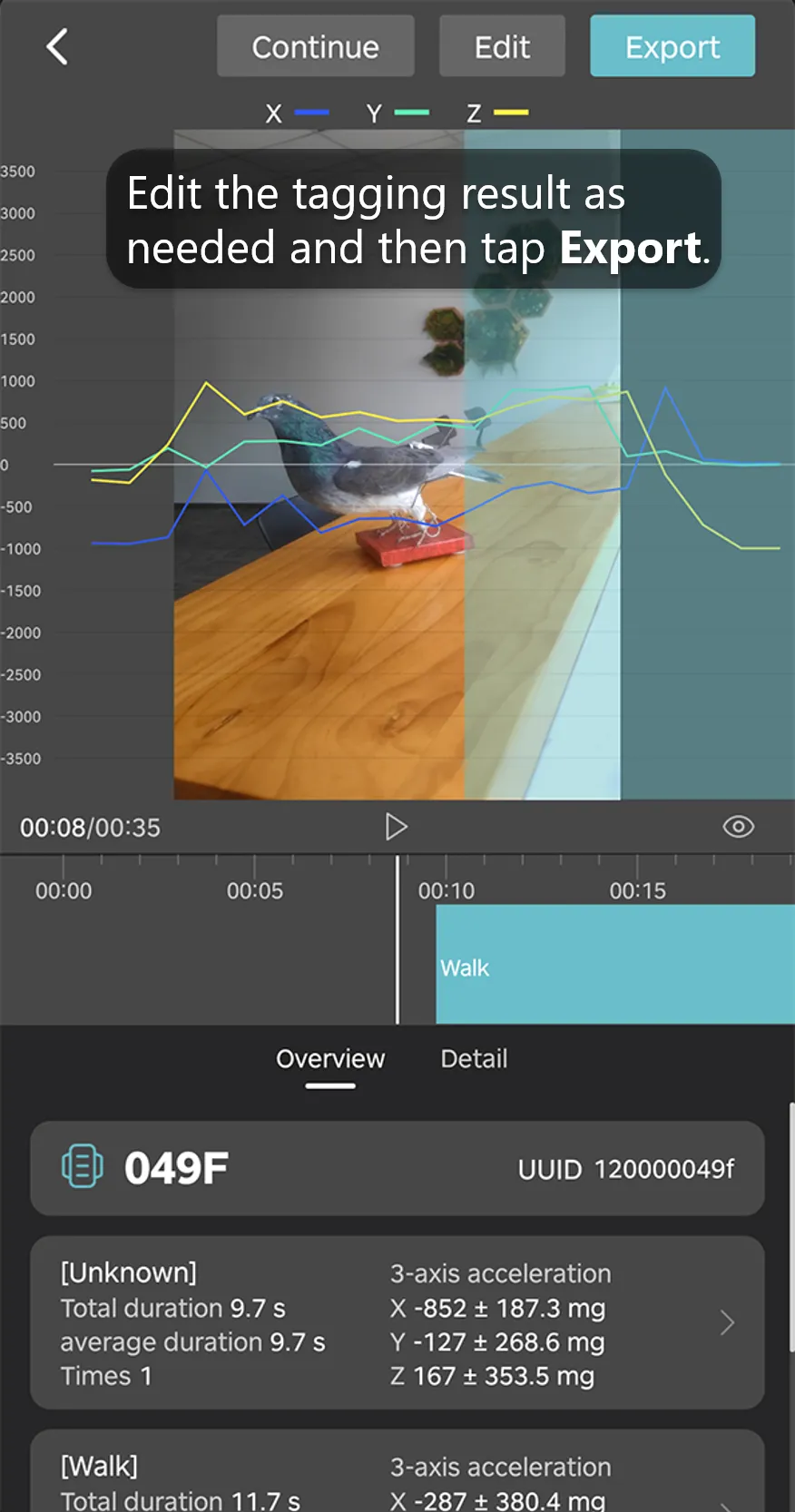
For more information on tag editing, see Editing tag result.
Step 7:
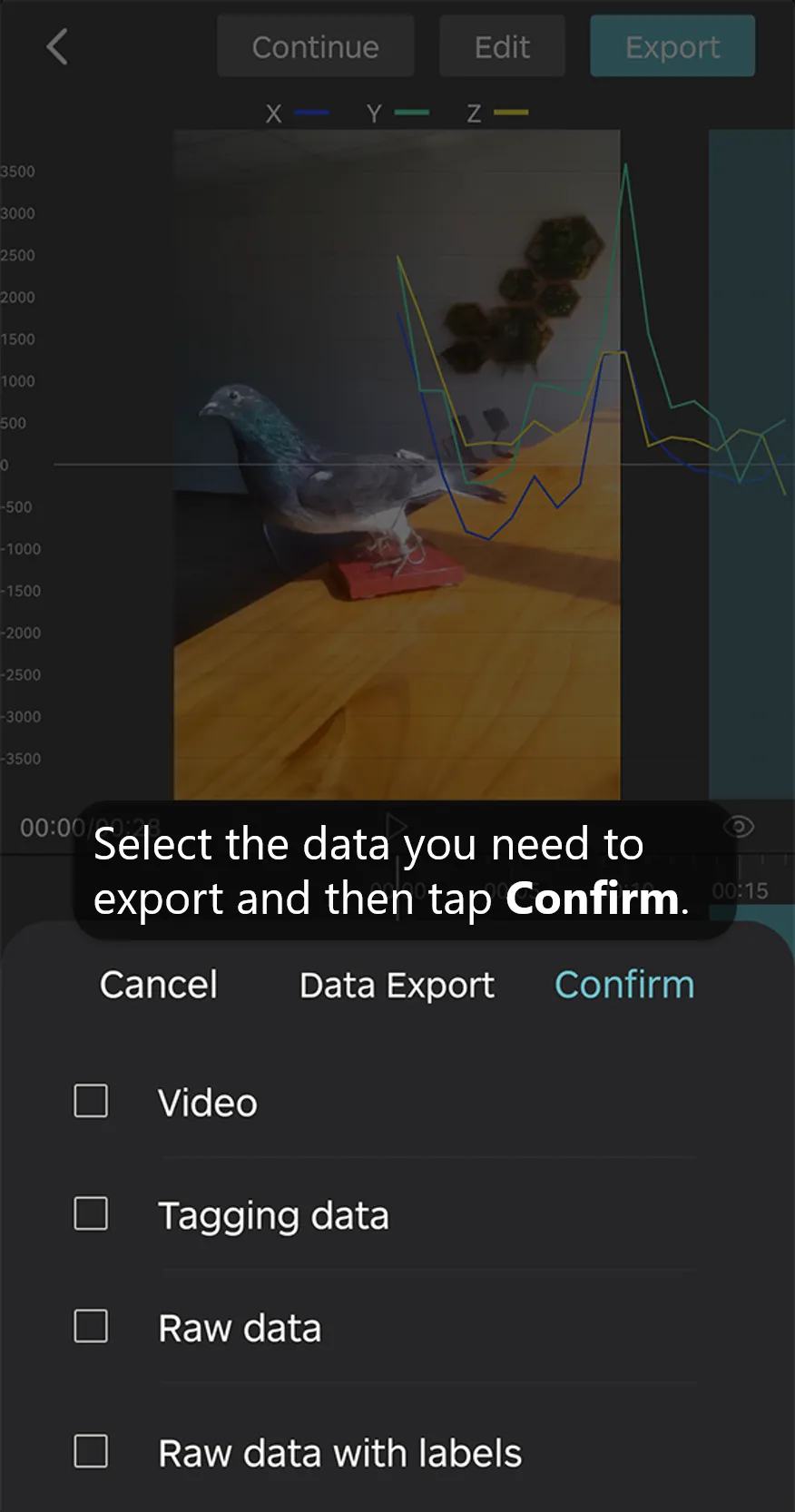
You can export the following data:
Video
Video captured during the data tagging- File format of Android: <UUID>[video]-<yyyy_mmdd_hhmm_ss_os3>.mp4
- File format of iOS: <UUID>-<yyyy_mmdd_hhmm_ss_os3>[Video].mp4
Tagging data
File with behavior label and timestamp- File format of Android: <UUID>[behavior]-<yyyy_mmdd_hhmm_ss_os3>.csv
- File format of iOS: <UUID>-<yyyymmdd_hhmmss_os3>[Behavior].csv
- Data field explanation:
- Start:Start time of a behavior
- End:End time of a behavior
- Tag:Selected label of a behavior
Raw data
ACC raw data collected during the data tagging- File format of Android: <UUID>[acc]-<yyyy_mmdd_hhmm_ss_os3>.csv
- File format of iOS: <UUID>-<yyyy_mmdd_hhmm_ss_os3>[Acc].csv
- Data field explanation:
- Collecting time: Time the device collects data, accurate to milliseconds
- X: Value of X-axis in 1/1024 g on the three-axis acceleration sensor
- Y: Value of Y-axis in 1/1024 g on the three-axis acceleration sensor
- Z: Value of Z-axis in 1/1024 g on the three-axis acceleration sensor
Note: The Collecting time exported by the Android system represents the duration elapsed since the beginning at each instance, measured in milliseconds and in numerical format. To facilitate your data processing, please refer to the code at the end to convert the Collecting time from milliseconds to complete timestamps.
Raw data with labels
ACC raw data with behavior label collected during the data tagging- File format of Android: <UUID>[acc+behavior]-<yyyy_mmdd_hhmm_ss_os3>.csv
- File format of iOS: <UUID>-<yyyy_mmdd_hhmm_ss_os3>[Behavior & Acc].csv
- Data field explanation:
- Collecting time: Time the device collects data, accurate to milliseconds
- X: Value of X-axis in 1/1024 g on the three-axis acceleration sensor
- Y: Value of Y-axis in 1/1024 g on the three-axis acceleration sensor
- Z: Value of Z-axis in 1/1024 g on the three-axis acceleration sensor
- Tag: Selected label of a behavior
Note: The Collecting time exported by the Android system represents the duration elapsed since the beginning at each instance, measured in milliseconds and in numerical format. To facilitate your data processing, please refer to the code at the end to convert the Collecting time from milliseconds to complete timestamps.
Please note that the time included in the file name and the time within the file content are both in the UTC+0 time zone.
After you export the data, you can tap User > Additional features > Data tagging to view the tagging history.
Please refer to the following R code to convert the Collecting time from milliseconds to complete timestamps.
# This Code Can Be Used To Process Accelerometer (acc) Or Accelerometer With Behavioral (acc+behavior) Data.
# However, These Two Types Of Data Cannot Be Processed Together And Need To Be Handled Separately.
# Make Sure You Have Installed The Stringr And Dplyr Packages
library(stringr)
library(dplyr)
# Place Your Files In The Corresponding Folder
folder_path <- "/Users/druid/Desktop/data_tagging/tag"
file_list <- list.files(folder_path, pattern = "*.csv", full.names = TRUE)
data_list <- lapply(file_list, function(file) {
file_data <- read.csv(file)
# Extracting ID and timestamp information
file_name <- basename(file)
file_parts <- unlist(str_split(file_name, "[-\\.]"))
UUID <- gsub("\\[.*\\]", "", file_parts[1]) # Remove [acc+behavior]
timestamp <- file_parts[2]
# Parsing timestamp information
year <- substr(timestamp, 1, 4)
month <- substr(timestamp, 6, 7)
day <- substr(timestamp, 8, 9)
hour <- substr(timestamp, 11, 12)
minute <- substr(timestamp, 13, 14)
second <- substr(timestamp, 16, 17)
millisecond <- substr(timestamp, 19, 21) # Extract millisecond part
# Formatting data into time format, UTC timezone
time_str <- paste(year, month, day, hour, minute, second, sep = "-")
time1 <- as.POSIXct(time_str, format = "%Y-%m-%d-%H-%M-%OS", tz = "UTC")
# Calculate time column, including milliseconds
file_data$time <- time1 + (file_data$Collecting.time + as.numeric(millisecond)) * 1e-3
file_data$time <- format(file_data$time, format = "%Y-%m-%d %H:%M:%OS3", tz = "UTC")
# Add ID and remove X.1 column
file_data$UUID <- UUID
file_data <- file_data[, -which(names(file_data) == "X.1")]
return(file_data)
})
# Due To Time Format Conversion In Calculations, The Results May Differ By 0.001 Seconds
tag_data <- bind_rows(data_list)
# Export File, You Can Customize The Filename
write.csv(tag_data, "tagging.csv")